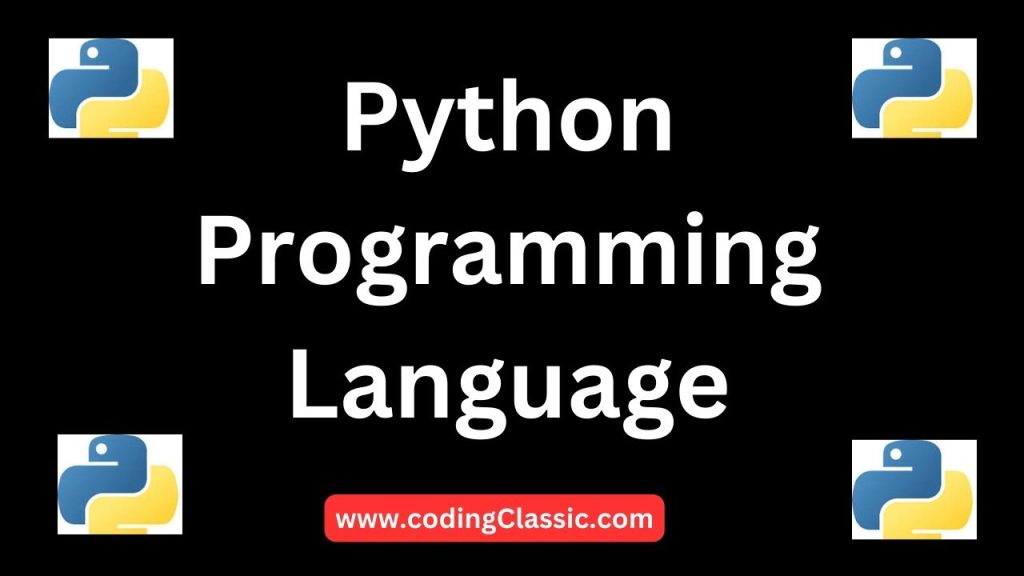
Question:
Haven’t been able to find a direct method for this anywhere. How can I add comments to a pull request via Azure Devops API in Python?
Answer:
If you want to add comments to a pull request via the Azure DevOps API in Python, you can use the requests
library to interact with the API. Here’s a step-by-step guide to help you with the process:
1. Install the necessary libraries:
If you don’t have the requests
library installed, you can install it using pip:
pip install requests
2. Generate a Personal Access Token (PAT):
You will need a PAT to authenticate your requests to the Azure DevOps API. You can generate a PAT from your Azure DevOps profile.
3. Use the Azure DevOps API to add comments to a pull request:
Below is a sample Python script that demonstrates how to add a comment to a pull request:
import requests
from requests.auth import HTTPBasicAuth
import json
# Define your Azure DevOps organization, project, repository, and pull request ID
organization = 'your_organization'
project = 'your_project'
repository = 'your_repository'
pull_request_id = 'your_pull_request_id'
# Personal Access Token (PAT)
personal_access_token = 'your_personal_access_token'
# URL for the pull request comments API
url = f'https://dev.azure.com/{organization}/{project}/_apis/git/repositories/{repository}/pullRequests/{pull_request_id}/threads?api-version=7.0'
# Comment content
comment_content = "This is a comment added via the Azure DevOps API."
# Payload for the API request
payload = {
"comments": [
{
"parentCommentId": 0,
"content": comment_content,
"commentType": 1
}
],
"status": 1
}
# Headers for the API request
headers = {
'Content-Type': 'application/json'
}
# Make the API request to add the comment
response = requests.post(url, headers=headers, auth=HTTPBasicAuth('', personal_access_token), data=json.dumps(payload))
# Check the response
if response.status_code == 201:
print("Comment added successfully!")
else:
print(f"Failed to add comment. Status code: {response.status_code}")
print(response.json())
In this script:
- Replace
your_organization
,your_project
,your_repository
,your_pull_request_id
, andyour_personal_access_token
with your actual Azure DevOps organization name, project name, repository name, pull request ID, and PAT. - The
url
variable constructs the API endpoint for adding comments to a specific pull request. - The
payload
variable contains the data to be sent in the request, including the comment content.
4. Run the script:
Execute the script to add the comment to the specified pull request.
Conclusion:
This script will send a POST request to the Azure DevOps API to add a comment to the specified pull request. If the request is successful, it will print a success message; otherwise, it will print an error message with the status code and response content.