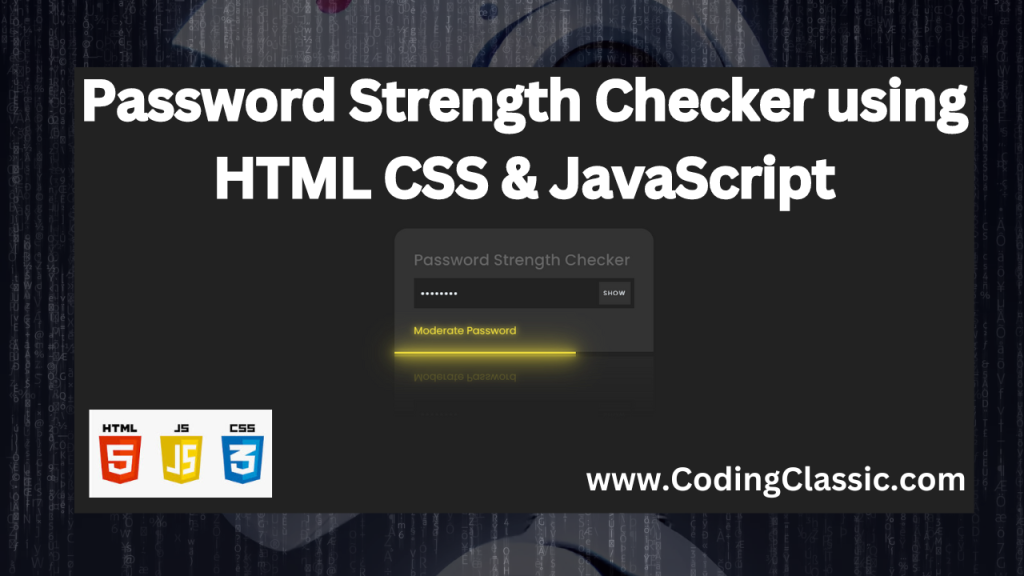
Password Strength Checker using HTML CSS and JavaScript
This project aims to create a simple yet effective password strength checker using HTML for structure, CSS for styling, and JavaScript for logic and interaction. The checker will analyze the user’s input and provide feedback on its strength, encouraging users to create secure passwords.
Introduction
Strong passwords are crucial for online security. A robust password checker helps users understand the strength of their chosen passwords and encourages them to create more secure ones. This project will demonstrate a basic implementation of such a checker, highlighting the key concepts involved.
1. HTML Structure: (index.html)
This HTML document builds a password strength checker. It features a heading, an input field for passwords, a toggle to show or hide the password, and a strength meter. The JavaScript file “script.js” analyzes the password’s strength and updates the meter accordingly. The CSS file “style.css” styles the elements for a visually appealing interface.
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Character encoding for the document -->
<meta charset="UTF-8">
<!-- Title of the webpage that appears in the browser tab -->
<title>Glowing Password Strength Checker</title>
<!-- Link to external CSS file for styling -->
<link rel="stylesheet" href="./style.css">
</head>
<body>
<!-- Container for the password strength checker -->
<div class="container">
<!-- Heading for the password strength checker -->
<h2>Password Strength Checker</h2>
<!-- Container for the password input field and show password toggle -->
<div class="inputArea">
<!-- Input field for password entry -->
<input type="password" placeholder=" Password" id="YourPassword">
<!-- Container for the show password toggle (e.g., an eye icon) -->
<div class="show"></div>
</div>
<!-- Container for displaying the password strength meter -->
<div class="strengthMeter"></div>
</div>
<!-- Link to external JavaScript file for functionality -->
<script src="./script.js"></script>
</body>
</html>
2. CSS Styling: (style.css)
This CSS code styles the password strength checker, creating a visually appealing and informative interface. The main container has a dark background, padding, and rounded corners for a modern look. The password input field is also dark with a borderless design for a clean appearance. The strength meter is placed at the bottom and dynamically changes its style based on the password’s strength, providing clear visual feedback. The show/hide password toggle is conveniently positioned in the top right corner. The CSS also includes specific styles for different strength levels, displaying appropriate messages to guide the user towards creating stronger passwords.
/* Import Google font 'Poppins' in various weights for use throughout the document */
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@100;200;300;400;500;600;700;800;900&display=swap");
/* Apply a reset and base styles to all elements */
* {
margin: 0; /* Remove default margin */
padding: 0; /* Remove default padding */
box-sizing: border-box; /* Include padding and border in element's total width and height */
font-family: "Poppins", sans-serif; /* Use Poppins font as the primary font */
}
body {
display: flex; /* Use flexbox layout */
align-items: center; /* Center items vertically */
justify-content: center; /* Center items horizontally */
min-height: 100vh; /* Ensure body takes up at least the full viewport height */
background: #222; /* Set a dark background color */
}
/* Style for the main container of the password strength checker */
.container {
position: relative; /* Position relative to allow absolute positioning of child elements */
width: 400px; /* Set a fixed width */
border-radius: 20px 20px 0 0; /* Round top corners */
padding: 30px; /* Add padding inside the container */
background: #333; /* Set a dark background color */
display: flex; /* Use flexbox layout */
justify-content: center; /* Center items horizontally */
/* align-items: center; */ /* Commented out; uncomment to center items vertically */
flex-direction: column; /* Stack child elements vertically */
border-radius: 1px solid #111; /* Incorrect syntax for border-radius, should use border instead */
gap: 10px; /* Space between child elements */
padding-bottom: 70px; /* Add padding at the bottom */
-webkit-box-reflect: below 1px linear-gradient(transparent, transparent, #0005); /* Add reflection effect for webkit browsers */
}
.container h2 {
color: #666; /* Set the text color */
font-weight: 500; /* Set the font weight */
}
/* Style for the input area */
.container .inputArea {
position: relative; /* Position relative to allow absolute positioning of child elements */
width: 100%; /* Full width of the container */
}
/* Style for the password input field */
.container .inputArea input {
position: relative; /* Position relative */
width: 100%; /* Full width of the input area */
background: #222; /* Dark background color */
border: none; /* Remove default border */
outline: none; /* Remove default outline */
padding: 10px; /* Add padding inside the input field */
color: aliceblue; /* Set text color */
font-size: 1.1em; /* Set font size */
}
/* Style for the strength meter */
.container .strengthMeter {
position: absolute; /* Position absolute within the container */
left: 0; /* Align to the left */
bottom: 0; /* Align to the bottom */
width: 100%; /* Full width of the container */
height: 3px; /* Set height */
background: #222; /* Dark background color */
}
/* Style for the strength meter's dynamic bar */
.container .strengthMeter::before {
content: ""; /* Empty content for the dynamic bar */
position: absolute; /* Position absolute within the strength meter */
width: 0; /* Start with width 0 */
height: 100%; /* Full height of the strength meter */
transition: 0.5s; /* Smooth transition for width change */
background: #f00; /* Default red color */
}
/* Styles for different password strength states */
.container.weak .strengthMeter::before {
width: 10%; /* Width for weak password */
background: #f00; /* Red color */
filter: drop-shadow(0 0 5px #f00) drop-shadow(0 0 10px #f00) drop-shadow(0 0 20px #f00); /* Red shadow effect */
}
.container.moderate .strengthMeter::before {
width: 70%; /* Width for moderate password */
background: #eedc3d; /* Yellow color */
filter: drop-shadow(0 0 5px #eedc3d) drop-shadow(0 0 10px #eedc3d) drop-shadow(0 0 20px #eedc3d); /* Yellow shadow effect */
}
.container.strong .strengthMeter::before {
width: 100%; /* Width for strong password */
background: #18e605; /* Green color */
filter: drop-shadow(0 0 5px #18e605) drop-shadow(0 0 10px #18e605) drop-shadow(0 0 20px #18e605); /* Green shadow effect */
}
/* Style for the password strength text */
.container .strengthMeter::after {
position: absolute; /* Position absolute within the strength meter */
top: -45px; /* Position above the strength meter */
left: 30px; /* Position from the left */
transition: 0.5s; /* Smooth transition for text change */
color: aliceblue; /* Set text color */
}
/* Styles for strength text based on password strength states */
.container.weak .strengthMeter::after {
content: "Weak Password"; /* Text for weak password */
color: #f00; /* Red color */
filter: drop-shadow(0 0 5px #f00); /* Red shadow effect */
}
.container.moderate .strengthMeter::after {
content: "Moderate Password"; /* Text for moderate password */
color: #eedc3d; /* Yellow color */
filter: drop-shadow(0 0 5px #eedc3d); /* Yellow shadow effect */
}
.container.strong .strengthMeter::after {
content: "Strong Password"; /* Text for strong password */
color: #18e605; /* Green color */
filter: drop-shadow(0 0 5px #18e605); /* Green shadow effect */
}
/* Style for the show/hide password button */
.show {
position: absolute; /* Position absolute within the container */
top: 0; /* Align to the top */
right: 0; /* Align to the right */
width: 60px; /* Fixed width */
height: 100%; /* Full height of the container */
background: #333; /* Dark background color */
border: 6px solid #222; /* Dark border */
cursor: pointer; /* Pointer cursor on hover */
justify-content: center; /* Center content horizontally */
align-items: center; /* Center content vertically */
display: flex; /* Use flexbox layout */
}
/* Style for the text in the show/hide button */
.show::before {
content: "Show"; /* Default text */
font-size: 0.6em; /* Smaller font size */
color: aliceblue; /* Text color */
letter-spacing: 0.15em; /* Space between letters */
text-transform: uppercase; /* Uppercase text */
}
/* Change text to 'Hide' when .hide class is added */
.show.hide::before {
content: "Hide"; /* Text for hiding password */
}
3. JavaScript Logic: ( script.js)
This code makes the password strength checker responsive to user input. It attaches an event listener to the password input field, triggering a strength check every time a key is released. The “Strength” function calculates the password’s strength, and based on the result, the container element’s class is updated. This class change dynamically alters the styling of the strength meter, providing visual feedback on the password’s security level.
/**
* Function to calculate the strength of the password based on different criteria.
* @param {string} password - The password to be evaluated.
* @returns {number} - A score representing the strength of the password.
*/
function Strength(password) {
let i = 0; // Initialize strength score
// Increment score for passwords longer than 6 characters
if (password.length > 6) {
i++;
}
// Increment score for passwords of length 10 or more
if (password.length >= 10) {
i++;
}
// Increment score if password contains at least one uppercase letter
if (/[A-Z]/.test(password)) {
i++;
}
// Increment score if password contains at least one number
if (/[0-9]/.test(password)) {
i++;
}
// Increment score if password contains at least one alphabetic letter or digit
// Note: The regular expression should be `[A-Za-z0-9]` instead of `[A-Za-z0-8]`
if (/[A-Za-z0-8]/.test(password)) {
i++;
}
return i; // Return the final strength score
}
// Select the container element
let container = document.querySelector(".container");
// Add an event listener to handle 'keyup' events
document.addEventListener("keyup", function (e) {
// Get the current value of the password input field
let password = document.querySelector("#YourPassword").value;
// Calculate the strength of the password
let strength = Strength(password);
// Apply appropriate classes based on the password strength
if (strength <= 2) {
container.classList.add("weak"); // Add 'weak' class for weak passwords
container.classList.remove("moderate"); // Remove 'moderate' class
container.classList.remove("strong"); // Remove 'strong' class
} else if (strength >= 2 && strength <= 4) {
container.classList.remove("weak"); // Remove 'weak' class
container.classList.add("moderate"); // Add 'moderate' class for moderate passwords
container.classList.remove("strong"); // Remove 'strong' class
} else {
container.classList.remove("weak"); // Remove 'weak' class
container.classList.remove("moderate"); // Remove 'moderate' class
container.classList.add("strong"); // Add 'strong' class for strong passwords
}
});
// Select the password input field and the show/hide button
let password = document.querySelector("#YourPassword");
let show = document.querySelector(".show");
// Add a click event listener to the show/hide button
show.onclick = function () {
// Toggle the type of the password input field between 'password' and 'text'
if (password.type === "password") {
password.setAttribute("type", "text"); // Show the password
show.classList.add("hide"); // Change button text to 'Hide'
} else {
password.setAttribute("type", "password"); // Hide the password
show.classList.remove("hide"); // Change button text to 'Show'
}
};
This JavaScript code handles the password strength calculation and updates the visual feedback:
- Gets references to the password input, strength bar, and strength text elements.
- Attaches an event listener to the input field, triggering the strength calculation whenever the password changes.
- The
calculatePasswordStrength
function evaluates the password based on length, character types, and complexity. - The
getStrengthText
function maps the calculated strength score to a descriptive text. - The strength bar width and text are updated based on the calculated strength.
Conclusion
This password strength checker provides a simple yet effective way to encourage users to create stronger passwords. By analyzing the password’s length, character types, and complexity, it provides visual and textual feedback, guiding users towards creating more secure passwords. This project can be further enhanced by incorporating more complex scoring algorithms and providing more detailed feedback to users.