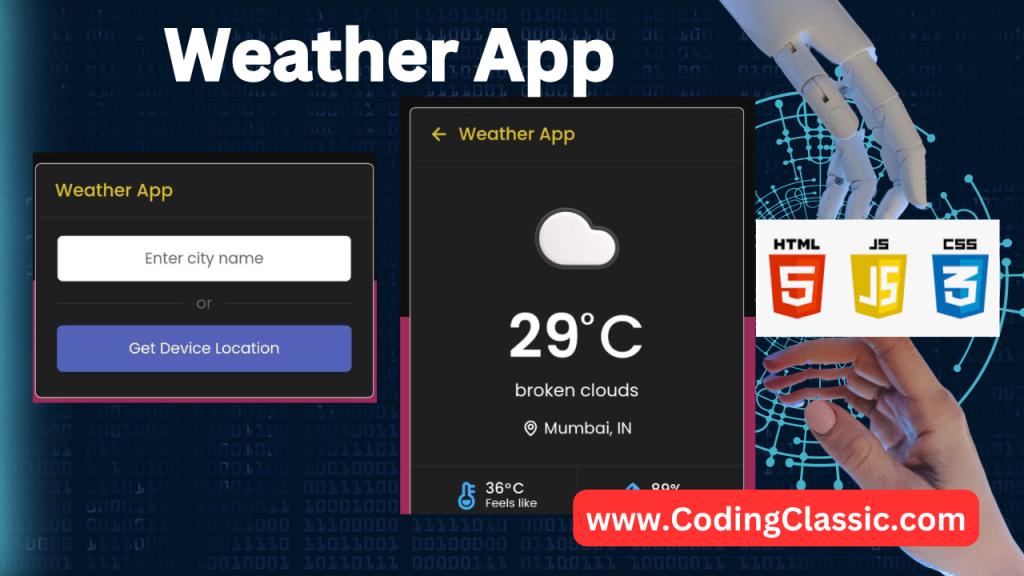
Let’s create a cool weather app together! We’ll use HTML to build the app’s structure, CSS to style it nicely, and JavaScript to make it interactive. No complicated stuff, just simple coding to get accurate weather information.
Building a weather app is a fantastic way to apply your HTML, CSS, and JavaScript skills to a real-world project. It’s a project that combines design, functionality, and data fetching, making it a comprehensive learning experience.
In this tutorial, we’ll guide you through the process of creating a basic weather app from scratch. We’ll start with the fundamental HTML structure, then enhance its appearance using CSS, and finally bring it to life with JavaScript to fetch and display weather data.
1. Project Setup and HTML Structure
This HTML document establishes the layout for a weather application, featuring distinct sections for user interaction, weather information, and supplementary details. The input area enables users to either enter a city name or utilize their device’s location services. The weather display section is designed to showcase various weather metrics, including temperature, conditions, location, perceived temperature, and humidity levels. The accompanying JavaScript file is expected to manage the retrieval and presentation of weather data, bringing the interface to life.
Here’s a breakdown of the HTML code with comments explaining each section:
2. CSS Styling: Enhancing the Look
This CSS code governs the visual appearance of a web page, specifying typography, background, color schemes, and layout configurations for diverse elements. It encompasses styling for input fields, buttons, weather displays, and other components, leveraging flexbox for layout management. Additionally, the code incorporates various aesthetic effects, such as box shadows and transitions, to enhance the overall user experience.
3. JavaScript Functionality: Bringing it to Life
This JavaScript code powers a weather application that enables users to retrieve weather information by entering a city name or using their device’s geolocation. The code establishes event listeners for user input and geolocation button clicks, and defines functions to send API requests to OpenWeatherMap, retrieve data, and display weather information. It also includes logic to handle various weather conditions, updating the UI with relevant icons, temperature, weather descriptions, location, and humidity details. Furthermore, the code incorporates error handling and a reset feature, allowing users to revert to the initial state.
Conclusion
Congratulations! You’ve successfully built a functional weather app using HTML, CSS, and JavaScript. You can now enter a city name, search for its weather, and view the temperature, description, and icon. This project demonstrates the power of web development and how you can leverage APIs to create interactive and useful applications.