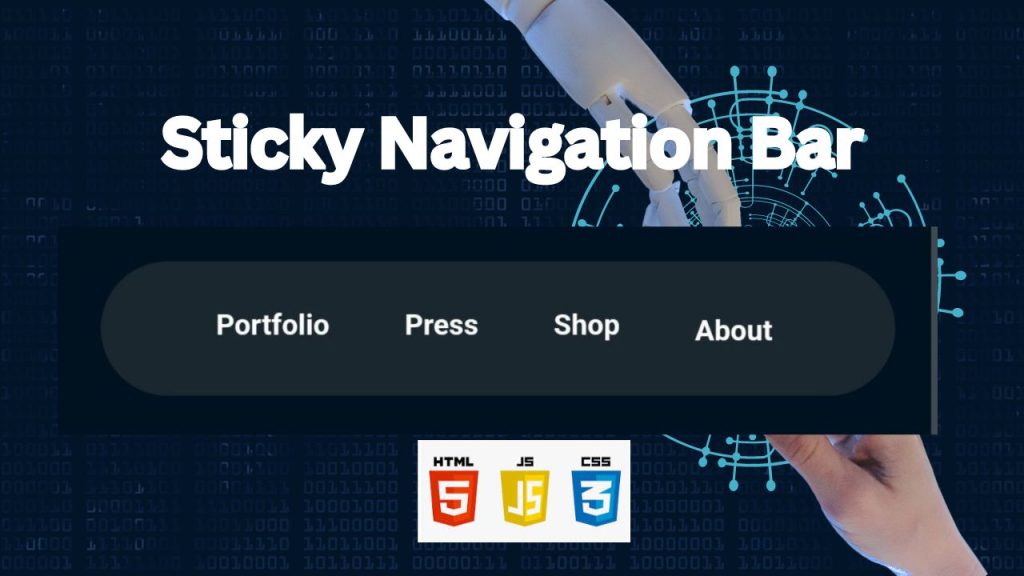
Introduction
A Fixed navigation bar, also known as a sticky or floating navbar, remains visible at the top of the webpage as users scroll down. This functionality enhances user experience by providing constant access to navigation links, which is particularly useful for long pages or content-heavy websites. In this tutorial, we’ll guide you through the steps to create a sticky navigation bar using HTML, CSS, and JavaScript. By the end, you’ll have a sleek and responsive navbar that sticks to the top of the viewport as users scroll.
1. HTML Structure: The Foundation
This HTML code creates a sticky navigation bar that allows users to navigate to different sections of the page. The navigation menu is contained within a header element and features links to various sections, each represented by a unique section element with an ID. The links use anchor tags with corresponding IDs as href attributes, enabling smooth scrolling to the respective sections. Additionally, the code incorporates a custom cursor effect that is achieved through a combination of JavaScript and CSS.
Here’s an explanation of the HTML code with comments:
2. Style the Navbar with CSS
This CSS code is responsible for styling the layout of a website, covering various elements such as the body, header, navigation, and sections. It also includes responsive design features through media queries, which adapt the layout to different screen sizes. Furthermore, the code defines background settings, a custom cursor animation, and animation effects classes, all of which contribute to a visually appealing and interactive user experience.
Here’s a breakdown of the CSS code with comments explaining each section:
3. JavaScript Functionality: Bringing it to Life
This JavaScript code snippet utilizes the Intersection Observer API to create a reusable class called IntersectionObserverList. This class enables developers to monitor when elements on a webpage intersect with a specified root element or the viewport. The class provides methods for adding, removing, and clearing observers for specific elements, allowing for flexible and efficient management of intersection observations. Additionally, the code includes a demonstration of how to leverage the IntersectionObserverList class to trigger animations when elements come into view, enhancing the user experience.
Certainly! Let’s break down the code step by step with comments.
Fantastic! We’ve successfully created a dynamic Sticky Navigation Bar using the power of HTML, CSS, and JavaScript. This means your website’s navigation will now seamlessly follow users as they scroll down the page. It’s a simple yet powerful feature that enhances user experience and makes your site more engaging. Well done!