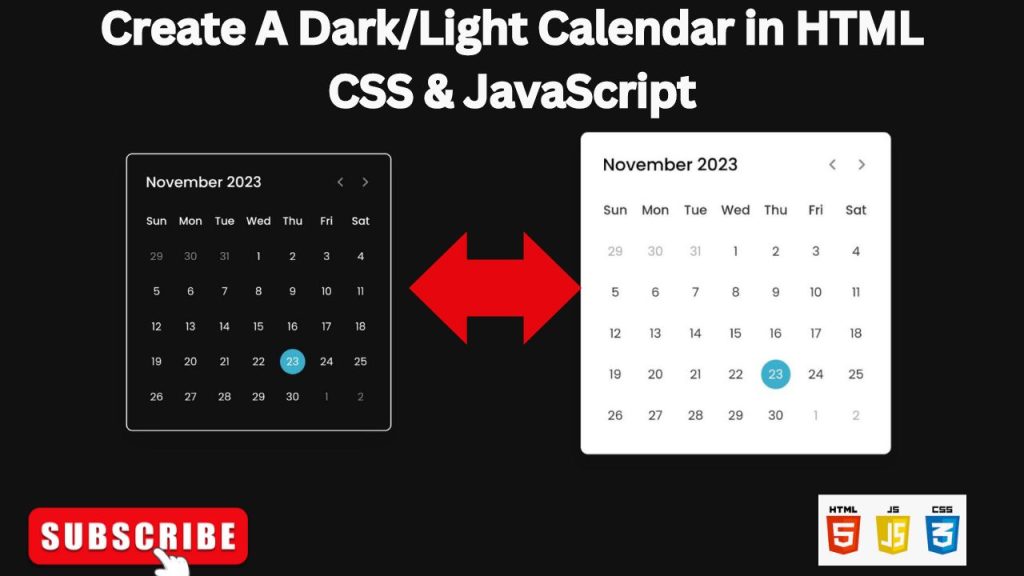
Get ready to embark on a fun and engaging project for beginners: building a Classic Calendar using HTML, CSS, and JavaScript. If you’re eager to explore more JavaScript projects, we’ve got you covered with a curated list of the top 10 projects suitable for beginners and intermediate learners, which you can check out for inspiration and learning.
A calendar is a useful tool that displays the days, weeks, and months of a year in a clear and organized manner. It’s a helpful resource for staying on top of important dates, such as holidays and special events, and keeping track of time.
In this tutorial, we’ll create a Classic calendar that not only displays the current date and day but also allows users to navigate through past, present, and future months using intuitive previous and next buttons. What’s even more impressive is that this calendar is built entirely with Vanilla JavaScript, eliminating the need for any external libraries or dependencies, making it a great example of JavaScript’s capabilities.
HTML Structure: The Calendar Grid
Create a new HTML file (e.g., index.html
) and add the following code:
CSS Styling: Light and Dark Themes
Create a new CSS file (e.g., style.css
) and add styles for both light and dark modes:
JAVASCRIPT :
Conclusion
You’ve now created a dynamic dark/light mode calendar using HTML, CSS, and JavaScript. You can customize the styling, add features like event highlighting, and integrate it with your website or application.