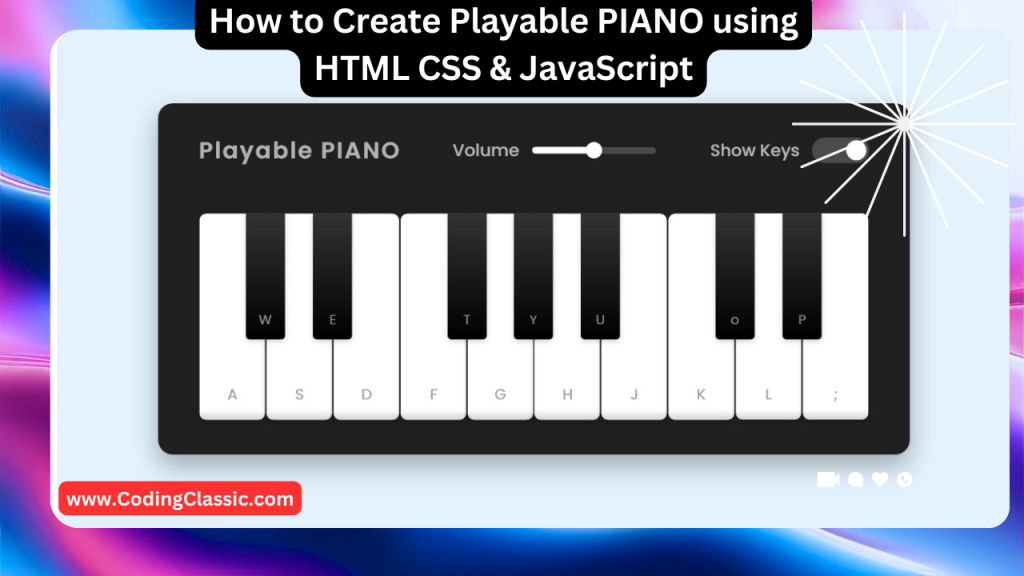
How to make Playable PIANO using HTML CSS & JavaScript
How to make Playable PIANO using HTML CSS & JavaScript
Introduction
This guide will walk you through creating a playable piano from scratch using HTML, CSS, and JavaScript. We’ll build a simple piano keyboard with keys that respond to clicks and play corresponding notes. This project combines the power of HTML for structure, CSS for styling, and JavaScript for interactivity to bring a musical instrument to life on the web.
1. HTML Structure (index.html):
This HTML code sets up the framework for a playable piano interface. It creates a main container with a header and a section for the piano keys. The header displays the title “Playable PIANO” and provides controls for adjusting volume and showing/hiding the keys.
The piano keys are organized within an unordered list, each key represented as a list item. Each key is visually categorized as either white or black, and linked to a specific keyboard key for triggering sound. The key letter is displayed within each key for visual reference. The styling and functionality are handled by separate CSS (style.css) and JavaScript (script.js) files, respectively.
2. CSS Styling (style.css):
This CSS code defines the visual appearance of a piano interface on a webpage. It incorporates the “Poppins” font from Google Fonts for a clean and modern look. The page layout is centered, with a background color, and the main piano interface container is styled with padding and a dark background.
The header is designed using flexbox to arrange the title and controls neatly. The volume slider and keys checkbox are styled for a visually appealing and user-friendly experience. The piano keys themselves are styled with different sizes and colors to distinguish between white and black keys.
The code includes media queries to adapt the layout for smaller screens, ensuring a responsive design by hiding certain keys and adjusting sizes for optimal viewing on various devices.
3. JavaScript Logic (script.js):
This JavaScript code brings a basic piano interface to life within a web page. It sets up the foundation for user interaction by identifying the piano keys, volume slider, and key visibility checkbox.
The code initializes a list of all piano keys and sets up a default audio tune. It then defines functions to play tunes, adjust the volume, show or hide the keys, and respond to keyboard presses.
Event listeners are added to trigger these functions based on user actions, such as clicking a key, adjusting the volume slider, checking the visibility checkbox, or pressing a key on the keyboard. This code creates a simple piano application where users can play tunes, control the volume, and toggle the visibility of the keys.
Conclusion:
This guide has provided a basic framework for building a playable piano from scratch. You can expand upon this foundation by adding features like:
- Keyboard Input: Allow users to play the piano using their computer keyboard.
- Visual Feedback: Display the note being played visually on the piano.
- Sound Effects: Add sound effects for key presses and releases.
- Multiple Instruments: Allow users to choose from different instruments.
With some creativity and coding skills, you can create a fun and interactive piano experience for users to enjoy.