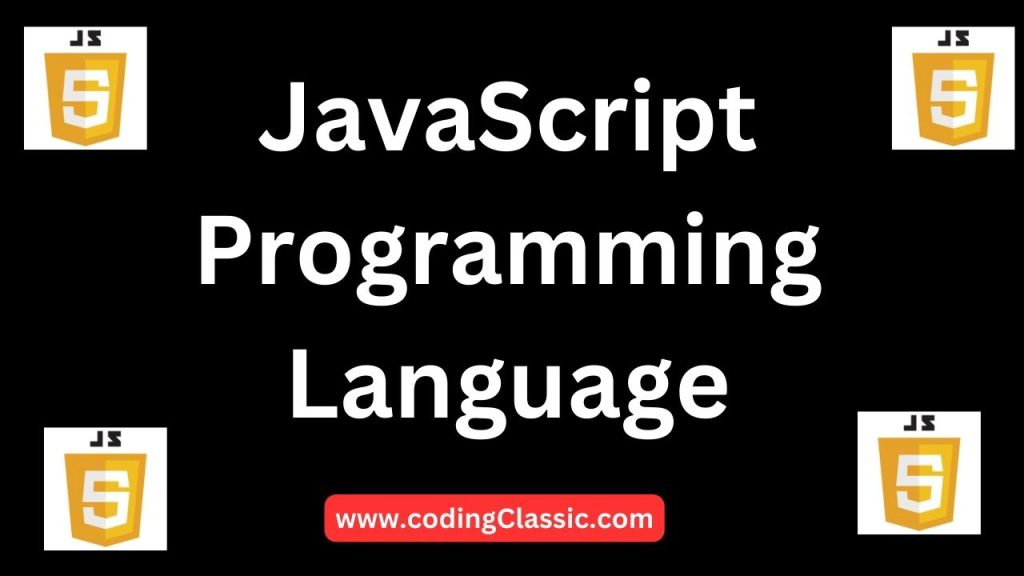
When building a WebRTC application with PeerJS, you may need to provide users with the ability to mute and unmute their microphone during a call. This can be achieved by manipulating the audio tracks of the user’s media stream. Here’s how you can implement this functionality.
How to Mute and Unmute a Microphone Using WebRTC and PeerJS
1. Set Up the Media Stream:
First, ensure you have obtained the user’s media stream, which includes audio and video tracks. This is typically done when the user joins a call.
navigator.mediaDevices.getUserMedia({ video: true, audio: true })
.then(stream => {
// Save the stream to a variable for later use
window.localStream = stream;
// Assuming you are using PeerJS to make a call
const peer = new Peer();
peer.on('call', call => {
call.answer(stream); // Answer the call with the local stream
});
})
.catch(error => {
console.error('Error accessing media devices.', error);
});
2. Mute the Microphone:
To mute the microphone, you need to disable the audio tracks in the media stream.
function muteMicrophone() {
if (window.localStream) {
window.localStream.getAudioTracks().forEach(track => {
track.enabled = false;
});
console.log('Microphone muted');
}
}
3. Unmute the Microphone:
Similarly, to unmute the microphone, you enable the audio tracks.
function unmuteMicrophone() {
if (window.localStream) {
window.localStream.getAudioTracks().forEach(track => {
track.enabled = true;
});
console.log('Microphone unmuted');
}
}
4. Attach Event Listeners:
You can attach these functions to buttons or other UI elements to allow users to mute and unmute their microphone during a call.
<button onclick="muteMicrophone()">Mute</button>
<button onclick="unmuteMicrophone()">Unmute</button>
Conclusion
By manipulating the audio tracks of the user’s media stream, you can easily implement mute and unmute functionality in a WebRTC application using PeerJS. This allows for a more interactive and user-friendly experience during video calls. Whether it’s for privacy, noise control, or any other reason, providing users with control over their microphone is an essential feature for modern web communication applications.