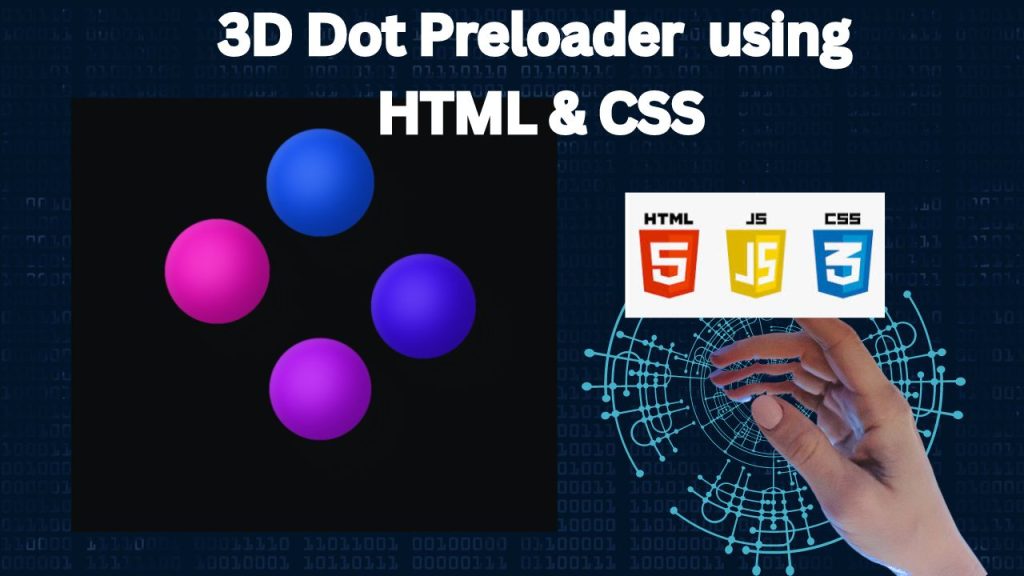
Let’s build a dynamic 3D dot loading animation using just HTML and CSS. We’ll create a visually captivating preloader by structuring it with HTML and bringing it to life with CSS animations. This project is perfect for honing your web design skills and adding a touch of flair to your website’s loading process. Let’s dive in and create an impressive loading experience!
HTML :
This HTML code establishes a simple webpage framework for a 3D dot preloader. It creates a basic structure using a div
container and four inner div
elements to represent the dots. The visual magic happens in the CSS file, where these elements will be styled and animated to form the 3D preloader effect.
CSS :
This CSS code brings the 3D dot preloader to life. It starts by resetting browser defaults and establishing color and animation variables. The preloader container and individual dots are styled with precise dimensions, positioning, and 3D transformations. Keyframe animations are meticulously crafted to produce the mesmerizing 3D rotation and movement of the dots, creating a captivating loading effect.
Conclusion:
Creating a 3D dot preloader with HTML and CSS is a rewarding project. By combining these fundamental web technologies, we’ve produced an eye-catching loading animation that enhances user experience. This endeavor is a great way to learn and apply web development skills to create dynamic and visually appealing website elements.