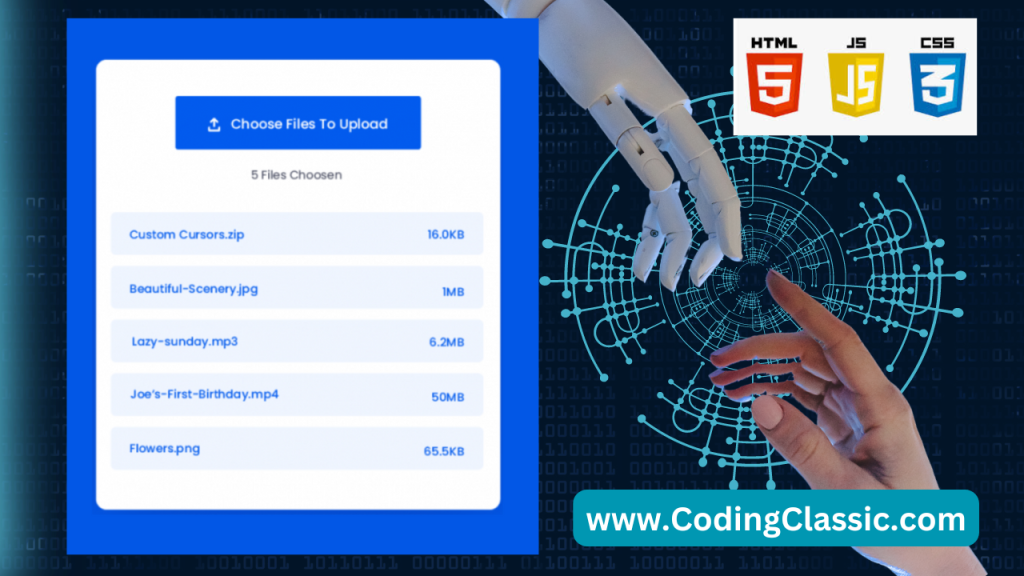
Beyond the Default: Crafting Custom File Upload Buttons with HTML, CSS, and JavaScript
The default file upload button in HTML is often bland and uninspired, failing to match the aesthetic of modern web designs. But fear not! With a little HTML, CSS, and JavaScript magic, you can transform that boring button into a visually appealing and user-friendly element.
This article will guide you through the process of creating custom file upload buttons, enhancing both their appearance and functionality.
1. The Foundation: HTML Structure
Start by creating a basic HTML structure for your custom button. This HTML code constructs a webpage featuring a customized file upload button. It leverages Font Awesome icons and Google Fonts for visual enhancements. The webpage’s central element is a container housing an input field for file selection, a custom button label, a display area for the number of selected files, and a list to showcase the chosen files.
2. Styling with CSS
This CSS defines the visual layout for the webpage. It configures the main container’s size, position, spacing, rounded corners, and shadow effect. The actual file input is concealed, while a label is formatted to serve as the visible upload button. Text showing the file count is given specific styling. The list of chosen files is customized with particular formatting and spacing between items.
3. JavaScript for Functionality
This JavaScript enhances the file input’s behavior. Upon file selection, it updates the interface to display the total count of chosen files. It also generates a list showing each selected file’s name and size. For files 1MB or larger, the size is presented in megabytes rather than kilobytes.
Conclusion
By combining HTML, CSS, and JavaScript, you can create custom file upload buttons that are both aesthetically pleasing and user-friendly. This allows you to enhance the user experience and seamlessly integrate file upload functionality into your web applications. Remember to experiment with different styles and functionalities to create buttons that perfectly match your website’s design and user needs.