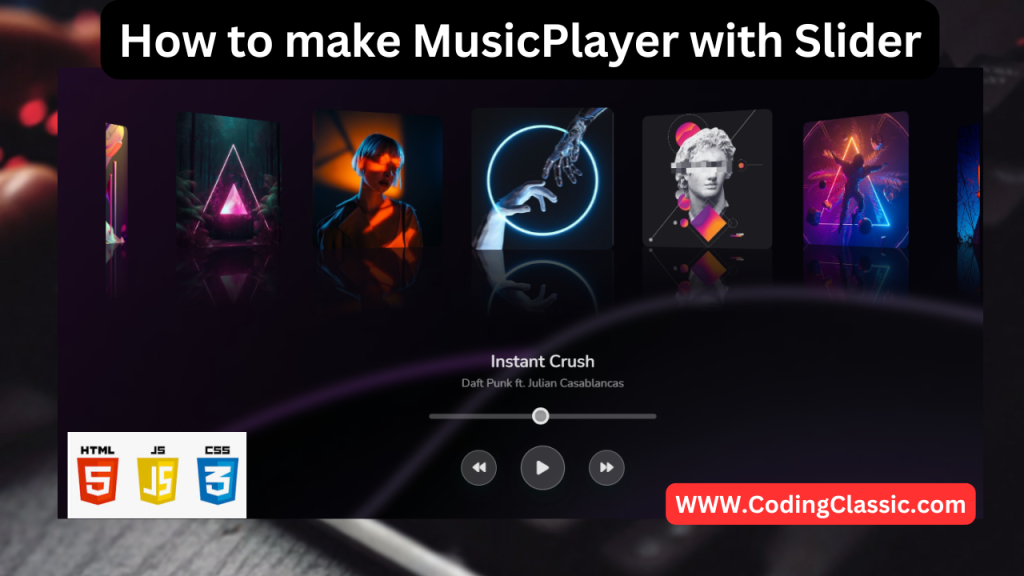
Building a Responsive Music Player with Slider using Swiper JS
This guide will walk you through creating a responsive music player with a slider feature using Swiper JS, HTML, CSS, and JavaScript. This project combines the power of Swiper JS for smooth slider functionality with the flexibility of HTML, CSS, and JavaScript for a visually appealing and interactive music player.
Introduction
The goal is to build a music player that adapts to different screen sizes and provides a user-friendly interface for navigating through a playlist. We’ll use Swiper JS to create a slider that displays the song titles and allows users to easily switch between tracks.
1. HTML (index.html):
This HTML code sets up a webpage with a music player that showcases album covers through a slider powered by the Swiper JS library. Each album cover serves as a link to a YouTube video. Beneath the slider, there is a music player equipped with play controls and a progress slider. Icons are provided by external libraries such as Font Awesome and Ionicons, while the functionality is managed by an external JavaScript file.
2. CSS Styling (style.css):
This CSS code styles a webpage with a focus on a music player and album display. It incorporates the Nunito font from Google Fonts for a clean and modern look. The background features a dynamic sliding animation, adding visual interest.
The album covers are showcased in a sleek Swiper slider, and when the user hovers over an album, a YouTube icon overlay appears, hinting at the possibility of watching music videos. The music player itself is designed with intuitive controls for playing, pausing, and navigating through tracks.
The progress slider indicates the current playback position, and the controls are enhanced with subtle hover effects and a slight scaling animation, providing a smooth and responsive user experience.
JavaScript Logic (script.js):
This JavaScript code is the engine behind a dynamic music player on a webpage. It acts as the brains, responding to user actions like playing, pausing, and switching between songs.
The code retrieves song information from a list and updates the player’s display to reflect the currently playing track. It uses carefully crafted functions to manage these actions and sets up event listeners to react to user clicks and interactions.
Furthermore, it seamlessly integrates with the Swiper library to create a visually appealing slider showcasing album covers, adding an extra layer of interactivity and enhancing the overall user experience.
Explanation of Code
- HTML Structure:
- The HTML code sets up the basic structure of the music player, including the player controls, progress bar, and a container for the Swiper JS slider.
- The
swiper-wrapper
will hold the song items dynamically added later.
- CSS Styling:
- The CSS provides basic styling for the music player components, ensuring a clean and visually appealing layout.
- Media queries are used to adjust the layout for different screen sizes, making the player responsive.
- JavaScript Logic:
- Song Data: The
songs
array holds information about each song, including title and artist. - Swiper JS Initialization: The Swiper JS instance is initialized, configuring the slider’s behavior (number of slides per view, spacing, pagination, breakpoints).
- Update Song Information: The
updateSongInfo()
function updates the song title and artist displayed in the player. - Play/Pause Functionality: The
playPause()
function toggles the playback state and updates the button text. - Previous/Next Song: The
prevSong()
andnextSong()
functions handle navigation to the previous and next songs in the playlist, respectively. - Update Progress Bar: The
updateProgressBar()
function updates the progress bar width based on the current playback time. - Event Listeners: Event listeners are attached to the buttons and the music player to handle user interactions and updates.
- Song Data: The
Conclusion
This guide has provided a comprehensive framework for building a responsive music player with a slider feature using Swiper JS, HTML, CSS, and JavaScript. You can customize this project further by adding features like volume control, shuffle, repeat, and more. The responsiveness of the player ensures a seamless user experience across various devices. Remember to replace the placeholder song data with your own music library to create a fully functional music player.