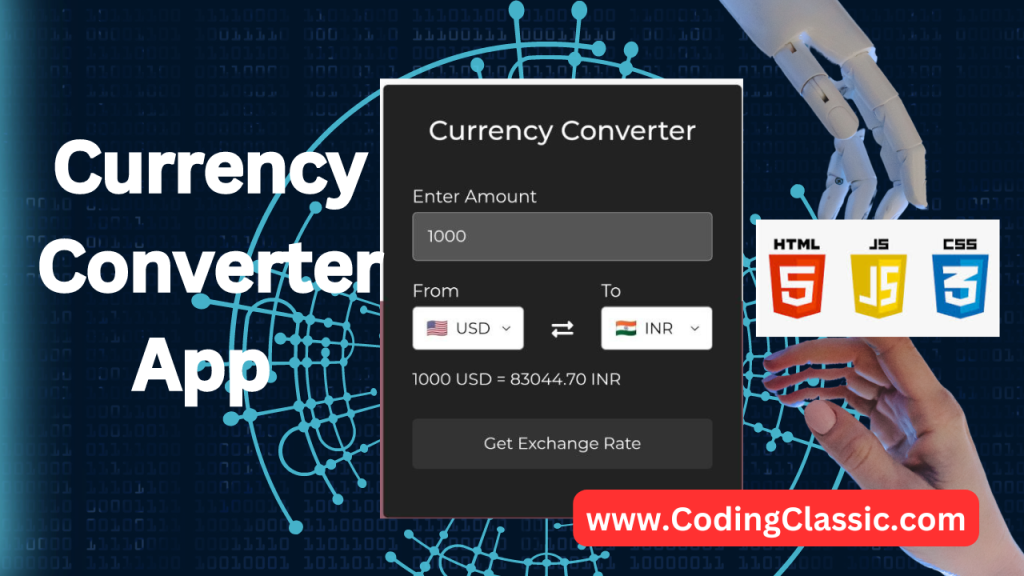
Welcome to today’s tutorial, where we’ll be building a practical and useful Currency Converter using HTML, CSS, and JavaScript. This project is perfect for coders of all levels, from beginners to enthusiasts, looking to add a valuable feature to their websites. We’ll use HTML to create the foundation, CSS to add some visual flair, and JavaScript to bring the conversion functionality to life. Join me on this coding adventure as we create a simple yet effective Currency Converter. Let’s dive in and get started on this fun and easy-to-follow project!
HTML :
This HTML file establishes the user interface for a currency converter, featuring input fields for specifying the amount to be converted, dropdown menus for selecting the original and target currencies, and a button to initiate the conversion process. The exchange rate is displayed in real-time. The accompanying JavaScript file, linked at the bottom of the HTML document, is expected to contain the logic for performing the currency conversions and making API requests to retrieve the necessary exchange rate data.
Here’s a breakdown of the HTML code with comments:
CSS :
This CSS code is responsible for visually styling a currency converter interface. It defines the layout and aesthetic for various elements, including input fields, dropdown menus, exchange rate displays, and buttons. The design features a sleek dark theme with bold color contrasts, and incorporates interactive effects such as hover and active states for the button. Additionally, the code employs the modern Montserrat font and a gradient background to create a visually appealing and cohesive design.
JavaScript:
This JavaScript code powers a currency exchange rate calculator, enabling users to convert between different currencies. It begins by defining a list of currency codes and their corresponding country codes, which are then used to dynamically populate two dropdown menus. Users can select the source and target currencies, enter an amount, and then retrieve the current exchange rate. The code utilizes an API to fetch the exchange rate data, using a provided API key for authentication, and then displays the result to the user in real-time.
Conclusion
Well done, coders! We’ve successfully completed our Currency Converter project using HTML, CSS, and JavaScript. This functional tool is now ready to be integrated into your projects, providing a practical solution for users. Regardless of whether you carefully followed each step or quickly skimmed through the tutorial, I hope you’ve gained valuable coding insights and experience that will benefit your future projects.