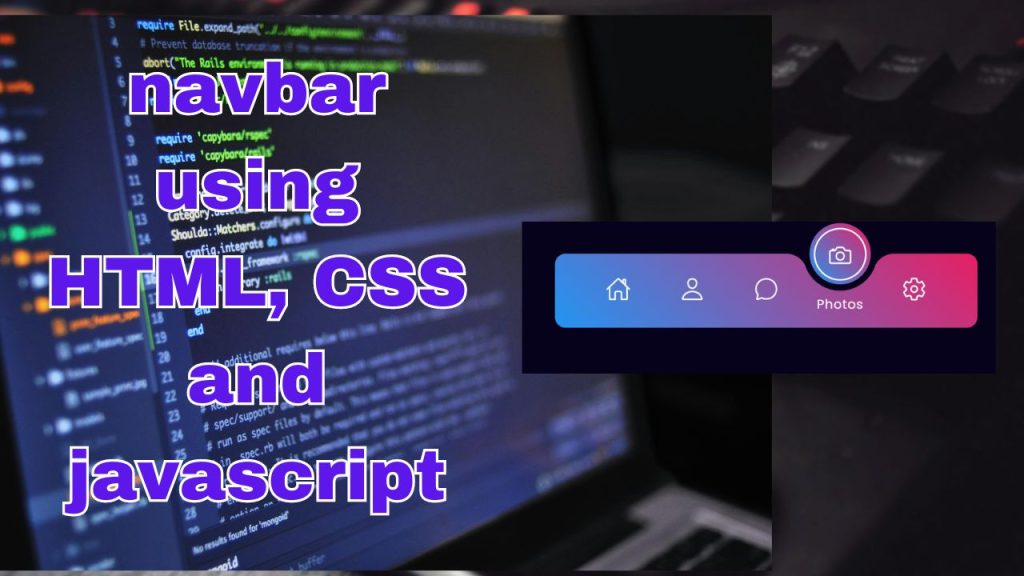
In the vast landscape of web development, one fundamental aspect reigns supreme: navigation. A seamless navigation experience can greatly enhance user interaction and accessibility on a website. Among the myriad of tools available to achieve this, the Navigation Menu Bar stands out as a cornerstone element. In this blog, we delve into the realm of web design, exploring how to create an elegant and functional Navigation Menu Bar using the trifecta of web technologies: HTML, CSS, and JavaScript. Join us on this journey as we unlock the secrets to crafting a captivating navigation experience that leaves users engaged and delighted.
So, let’s delve into the world of Magic Navigation Menu Indicators. Ever wondered what exactly constitutes a “magic navigation menu indicator”? Think of it as the dazzle in your web navigation. Essentially, it’s a dynamic, animated element of the menu that indicates your current position on a website. Its primary goal? To enhance the interactivity of the menu and showcase some impressive web enchantment.
The real magic happens when this indicator springs to life with animations. Instead of remaining static, when you click on a menu item, it undergoes a transformation – it might change colors, shift position, or add some captivating effects to signify that it’s the active selection. It’s these captivating animations that truly elevate the navigation experience on your website.
HTML Code (index.html)
CSS Code (style.css)
JavaScript Code (action.js)
This JavaScript code sets up event listeners on all elements with the class ‘list’. When a list item is clicked, it triggers the activeLink
function, which removes the ‘active’ class from all list items and then adds the ‘active’ class to the clicked list item. This effectively toggles the ‘active’ class between list items when they are clicked.
Conclusion
In the realm of web design, dynamic navigation menu indicators with active tab animations are a game-changer for enhancing the online experience. They’re not just mere guides; they act as companions throughout your web journey. These interactive elements not only assist in navigation but also maintain your engagement and provide immediate feedback, instilling a sense of empowerment.
As the landscape of web design progresses, we anticipate witnessing even more innovative applications of these magical navigation menu indicators. They wield the power to transform websites into engaging, user-friendly platforms, adding an extra layer of excitement to your online ventures. So, stay vigilant for these enchanting elements as they continue to weave their magic across the digital realm, turning your browsing experiences into something truly captivating.