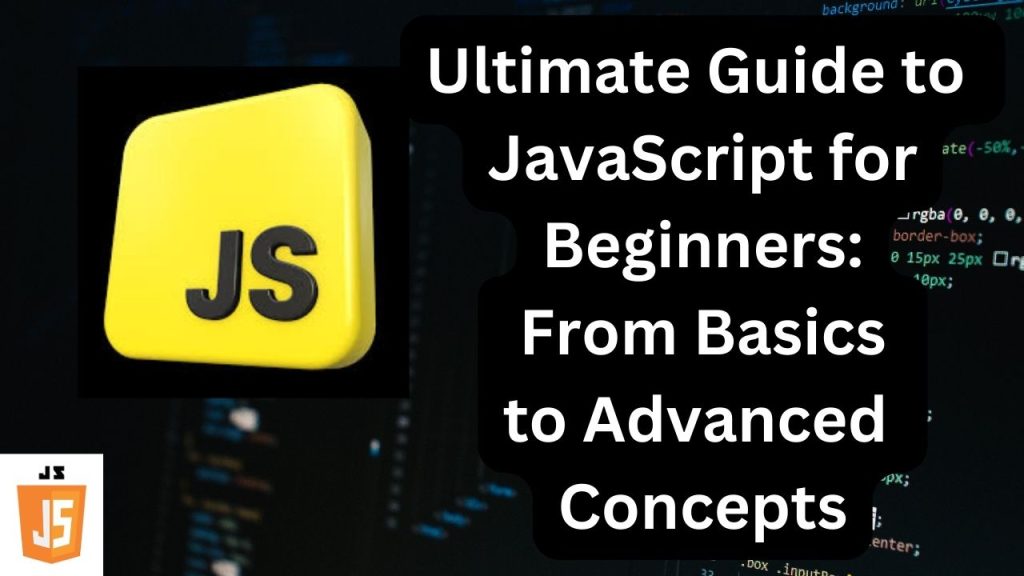
TABLE OF CONTENT
Introduction
- Brief overview of JavaScript
- Importance of JavaScript in web development
- Goals of the guide
Getting Started with JavaScript
- What is JavaScript?
- History and evolution of JavaScript
- Setting up the development environment
- Installing a text editor (e.g., VS Code)
- Running JavaScript in the browser
- Introduction to the browser console
JavaScript Basics
- Syntax and Structure
- Comments in JavaScript
- Semicolons and line breaks
- Variables and Data Types
var
,let
, andconst
- Primitive data types (string, number, boolean, null, undefined, symbol)
- Type conversion and coercion
- Operators
- Arithmetic operators
- Comparison operators
- Logical operators
- Control Structures
- Conditional statements (
if
,else
,switch
) - Loops (
for
,while
,do...while
)
- Conditional statements (
Functions and Scope
- Function Declaration and Expression
- Basic function syntax
- Function parameters and return values
- Arrow Functions
- Scope and Closures
- Global vs. local scope
- Lexical scoping and closures
Objects and Arrays
- Working with Objects
- Object literals and properties
- Methods and
this
keyword
- Arrays
- Array creation and manipulation
- Common array methods (
push
,pop
,map
,filter
,reduce
)
Introduction to the DOM
- What is the DOM?
- Selecting and Manipulating DOM Elements
document.getElementById
,document.querySelector
- Modifying element content and attributes
- Event Handling
- Adding event listeners
- Common events (click, submit, load)
Asynchronous JavaScript
- Callbacks
- Promises
- Creating and using promises
- Chaining and error handling
- Async/Await
- Syntax and usage
- Error handling with
try
/catch
Advanced JavaScript Concepts
- Prototypes and Inheritance
- The
this
Keyword and Context - Modules and Import/Export
- JavaScript ES6+ Features
- Template literals
- Destructuring
- Spread and rest operators
Debugging and Testing
- Common JavaScript Errors and How to Fix Them
- Using Browser Developer Tools
- Introduction to Testing Frameworks (e.g., Jest)
Best Practices and Tips
- Writing Clean and Maintainable Code
- Code Style Guidelines
- Performance Optimisation Tips
Resources and Further Learning
- Recommended Books and Online Courses
- JavaScript Communities and Forums
- Documentation and Reference Sites
Conclusion
- Recap of key points
- Encouragement to practice and explore
- Next steps in the learning journey
INTRODUCTION
JavaScript is a cornerstone of modern web development, enabling interactive and dynamic features on websites and applications. From simple web page manipulations to complex server-side logic, JavaScript’s versatility and widespread use make it an essential skill for developers. This guide aims to provide a thorough understanding of JavaScript, from fundamental concepts to advanced techniques, helping beginners build a solid foundation and advance their skills.
1. Getting Started with JavaScript
What is JavaScript?
JavaScript is a high-level, interpreted programming language mainly employed to generate dynamic and interactive web content. Created by Brendan Eich in 1995, it has grown from a basic scripting language into a robust resource for web development. JavaScript allows for the manipulation of web page elements, event handling, and server communication, establishing it as an essential component of contemporary web applications.
History and Evolution of JavaScript
JavaScript began as a scripting language for enhancing the interactivity of web pages. Over the years, it has undergone significant changes, with ECMAScript (ES) versions introducing new features and capabilities. Key milestones include:
- ECMAScript 3 (1999): Introduced regular expressions, try/catch, and better string handling.
- ECMAScript 5 (2009): Added features like strict mode, JSON support, and new array methods.
- ECMAScript 6 (2015): Brought significant updates, including arrow functions, classes, and template literals.
- ECMAScript 2016+: Continued evolution with features like async/await, modules, and new array methods.
Setting Up the Development Environment
Before diving into coding, you need to set up your development environment. Here’s how:
- Installing a Text Editor: Choose a text editor like Visual Studio Code, Sublime Text, or Atom. Visual Studio Code is highly recommended for its features and extensions.
- Running JavaScript in the Browser: Modern browsers have built-in JavaScript engines. Open the developer tools (usually by pressing F12) to access the console, where you can execute JavaScript code.
- Introduction to the Browser Console: The console allows you to test and debug JavaScript code. It provides real-time feedback and is essential for development and troubleshooting.
2. JavaScript Basics
Syntax and Structure
JavaScript syntax is the set of rules that define a correctly structured JavaScript program. Key aspects include:
- Comments: Use
//
for single-line comments and/* */
for multi-line comments. - Semicolons and Line Breaks: Semicolons (
;
) are used to terminate statements, but JavaScript often allows line breaks to separate statements.
// This is a single-line comment
var x = 10; // Semicolon to end the statement
/*
This is a
multi-line comment
*/
Variables and Data Types
Variables store data that can be used and manipulated throughout your program. JavaScript uses three keywords to declare variables:
var
: The traditional keyword for declaring variables. It has function scope.let
: Introduced in ES6, it has block scope and allows re-assignment.const
: Also introduced in ES6, it has block scope and is used for constants.
JavaScript supports various data types:
- Primitive Data Types: Include
string
,number
,boolean
,null
,undefined
, andsymbol
. - Type Conversion and Coercion: JavaScript automatically converts data types in certain situations (e.g., adding a number to a string results in a string).
let number = 10;
let text = "The number is " + number; // Type coercion
let str = "5";
let num = Number(str); // Type conversion from string to number
Operators
Operators perform operations on variables and values. Key types include:
- Arithmetic Operators:
+
,-
,*
,/
,%
- Comparison Operators:
==
,===
,!=
,!==
,>
,<
,>=
,<=
- Logical Operators:
&&
(AND),||
(OR),!
(NOT)
let a = 5;
let b = 10;
console.log(a + b); // Arithmetic: 15
console.log(a === b); // Comparison: false
console.log(a < b && b < 20); // Logical: true
Control Structures
Control structures dictate the flow of your program:
- Conditional Statements: Use
if
,else if
, andelse
to execute code based on conditions.
let age = 20;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
- Loops: Allow repeated execution of code blocks. Types include
for
,while
, anddo...while
.
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs 0 through 4
}
let j = 0;
while (j < 5) {
console.log(j); // Outputs 0 through 4
j++;
}
3. Functions and Scope
Function Declaration and Expression
Functions are blocks of code designed to perform a particular task. They can be declared or expressed:
- Function Declaration: A named function defined using the
function
keyword.
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice")); // Outputs: Hello, Alice
- Function Expression: An anonymous function assigned to a variable.
let multiply = function(x, y) {
return x * y;
};
console.log(multiply(4, 5)); // Outputs: 20
Arrow Functions
Introduced in ES6, arrow functions offer a shorter syntax and lexically bind the this
value.
- Syntax:
const add = (x, y) => x + y;
console.log(add(3, 4)); // Outputs: 7
Scope and Closures
- Scope: Refers to the accessibility of variables. JavaScript has global scope and function (or local) scope.
let
andconst
have block scope.
function example() {
let localVar = "I am local";
console.log(localVar); // Accessible here
}
console.log(localVar); // Error: localVar is not defined
- Closures: Functions that retain access to their lexical scope even when the function is executed outside that scope.
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Outputs: 1
console.log(counter()); // Outputs: 2
4. Objects and Arrays
Working with Objects
Objects are collections of key-value pairs. They are fundamental in JavaScript for storing and manipulating data.
- Object Literals:
let person = {
name: "John",
age: 30,
greet: function() {
return "Hello, " + this.name;
}
};
console.log(person.name); // Outputs: John
console.log(person.greet()); // Outputs: Hello, John
- Methods and
this
Keyword:this
refers to the object from which a method was called.
let car = {
brand: "Toyota",
model: "Corolla",
getDetails: function() {
return this.brand + " " + this.model;
}
};
console.log(car.getDetails()); // Outputs: Toyota Corolla
Arrays
Arrays store ordered collections of data. They are essential for managing lists of items.
- Array Creation and Manipulation:
let fruits = ["apple", "banana", "cherry"];
fruits.push("date"); // Add an item
console.log(fruits); // Outputs: ["apple", "banana", "cherry", "date"]
- Common Array Methods:
let numbers = [1, 2, 3, 4, 5];
let squared = numbers.map(num => num * num); // [1, 4, 9, 16, 25]
let evenNumbers = numbers.filter(num => num % 2 === 0); // [2, 4]
let sum = numbers.reduce((acc, num) => acc + num, 0); // 15
5. Introduction to the DOM
What is the DOM?
The Document Object Model (DOM) represents the structure of an HTML document as a tree of objects. It allows JavaScript to interact with and manipulate web page content.
Selecting and Manipulating DOM Elements
- Selecting Elements:
let element = document.getElementById("myElement");
let anotherElement = document.querySelector(".myClass");
- Modifying Content and Attributes:
element.textContent = "New text content";
element.setAttribute("data-info", "some data");
Event Handling
Events are actions or occurrences (e.g., clicks, form submissions) that JavaScript can respond to.
- Adding Event Listeners:
element.addEventListener("click", function() {
alert("Element clicked!");
});
- Common Events:
let button = document.querySelector("button");
button.addEventListener("click", function() {
console.log("Button was clicked!");
});
window.addEventListener("load", function() {
console.log("Page loaded");
});
6. Asynchronous JavaScript
Callbacks
Callbacks are functions passed as arguments to other functions, executed after an operation completes.
- Example:
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched");
}, 2000);
}
fetchData(function(data) {
console.log(data); // Outputs: Data fetched
});
Promises
Promises represent a value that may be available now, or in the future, or never. They handle asynchronous operations more gracefully than callbacks.
- Creating and Using Promises:
let promise = new Promise((resolve, reject) => {
let success = true;
if (success) {
resolve("Promise resolved!");
} else {
reject("Promise rejected!");
}
});
promise.then(result => {
console.log(result); // Outputs: Promise resolved!
}).catch(error => {
console.error(error);
});
- Chaining and Error Handling:
fetchData()
.then(data => processData(data))
.then(result => console.log(result))
.catch(error => console.error("Error:", error));
Async/Await
Async/Await syntax allows writing asynchronous code that looks synchronous, making it easier to read and manage.
- Syntax and Usage:
async function fetchData() {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
return data;
}
fetchData().then(data => console.log(data));
- Error Handling:
async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
return data;
} catch (error) {
console.error("Error:", error);
}
}
7. Advanced JavaScript Concepts
Prototypes and Inheritance
JavaScript uses prototypes to enable inheritance. Every object has a prototype from which it can inherit properties and methods.
- Example:
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
return "Hello, " + this.name;
};
let alice = new Person("Alice");
console.log(alice.greet()); // Outputs: Hello, Alice
The this
Keyword and Context
The this
keyword refers to the object that the function is a property of. Its value depends on how the function is called.
- Example:
let car = {
brand: "Toyota",
getBrand: function() {
return this.brand;
}
};
console.log(car.getBrand()); // Outputs: Toyota
Modules and Import/Export
JavaScript modules allow you to break code into reusable pieces. Use import
and export
to manage dependencies.
- Exporting Modules:
// math.js
export function add(a, b) {
return a + b;
}
- Importing Modules:
// app.js
import { add } from './math.js';
console.log(add(2, 3)); // Outputs: 5
JavaScript ES6+ Features
- Template Literals:
let name = "John";
let greeting = `Hello, ${name}!`;
- Destructuring:
let person = { name: "Alice", age: 25 };
let { name, age } = person;
- Spread and Rest Operators:
let numbers = [1, 2, 3];
let moreNumbers = [...numbers, 4, 5];
function sum(...args) {
return args.reduce((acc, num) => acc + num, 0);
}
8. Debugging and Testing
Common JavaScript Errors and How to Fix Them
- Syntax Errors: Mistakes in code syntax. Check for missing semicolons or brackets.
- Reference Errors: Trying to access undefined variables. Ensure variables are declared before use.
- Type Errors: Performing operations on incompatible types. Verify variable types and operations.
Using Browser Developer Tools
- Console: Monitor outputs, errors, and logs.
- Debugger: Set breakpoints and step through code to inspect variables and control flow.
Introduction to Testing Frameworks
- Jest: A popular testing framework for JavaScript, providing a simple API for writing and running tests.
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
9. Best Practices and Tips
Writing Clean and Maintainable Code
- Use Meaningful Variable Names: Choose descriptive names for variables and functions.
- Avoid Global Variables: Limit the use of global variables to prevent conflicts.
- Modularize Code: Break code into functions and modules for better organization.
Code Style Guidelines
- Consistent Indentation: Use spaces or tabs consistently.
- Commenting: Add comments to explain complex logic.
- Follow Style Guides: Adhere to style guides like Airbnb or Google for consistency.
Performance Optimization Tips
- Minimize DOM Manipulations: Batch updates to reduce reflows and repaints.
- Use Efficient Algorithms: Optimize algorithms for better performance.
- Leverage Caching: Cache frequently used data to reduce computation time.
10. Resources and Further Learning
Recommended Books and Online Courses
- Books:
- “Eloquent JavaScript” by Marijn Haverbeke
- “JavaScript: The Good Parts” by Douglas Crockford
- Online Courses:
- Codecademy’s JavaScript course
- freeCodeCamp’s JavaScript Algorithms and Data Structures
JavaScript Communities and Forums
- Communities:
- Stack Overflow
- Reddit’s r/javascript
- Forums:
- JavaScript Weekly
- MDN Web Docs community
Documentation and Reference Sites
- MDN Web Docs: Comprehensive resource for JavaScript and web technologies.
- JavaScript.info: Detailed tutorials and explanations of JavaScript concepts.
Conclusion
In this guide, we’ve explored JavaScript from its basic syntax to advanced concepts. Understanding these fundamentals equips you with the skills to build interactive web applications and solve complex problems. Continue practicing and experimenting with JavaScript to deepen your knowledge and stay updated with the latest features and best practices. Happy coding!