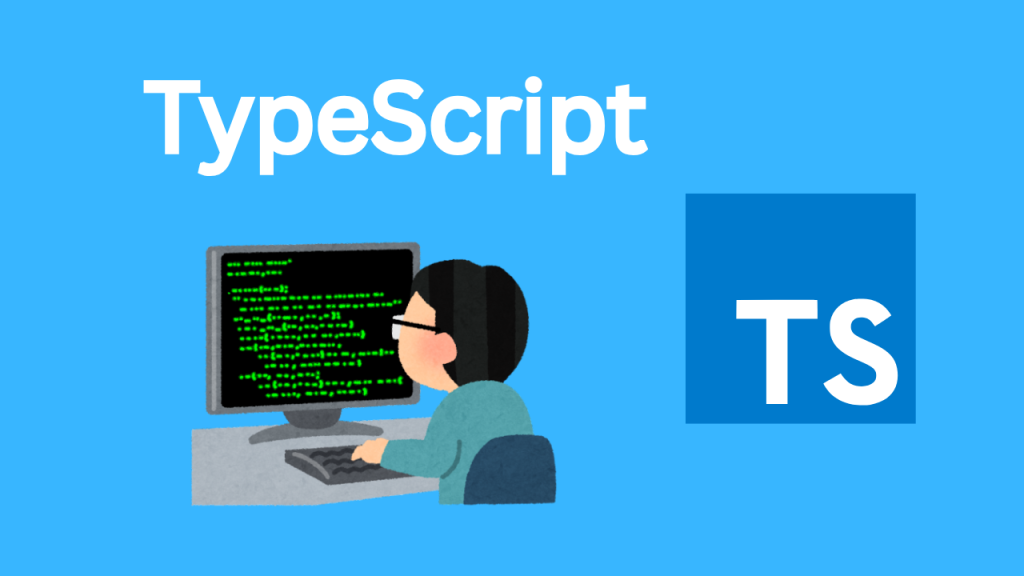
Troubleshooting Vitest: How to Mock the hashSync
Function in TypeScript
Vitest is a powerful testing framework designed for modern JavaScript and TypeScript applications. However, when dealing with complex dependencies such as hashing functions, mocking can sometimes be challenging. One common issue arises when attempting to mock the hashSync
function in libraries like bcrypt.
In this article, we’ll explore how to resolve this problem effectively, ensuring your tests run as expected.
Understanding the Problem
Hashing functions like hashSync
are deterministic and non-reversible, making them ideal for securely storing sensitive data like passwords. These functions are typically provided by libraries such as bcrypt or crypto.
When testing, you might want to mock these functions to avoid running the actual hashing logic, which can be computationally expensive. Unfortunately, mocking hashSync
isn’t always straightforward, especially when using Vitest in a TypeScript project.
Scenario: Mocking hashSync
Consider the following scenario:
- You have two methods for handling hashing and comparison:
hashPassword
: hashes a plaintext password.comparePassword
: compares a plaintext password with a hash.
- These methods rely on
hashSync
andcompareSync
from a library like bcrypt.
Here’s an example implementation:
import bcrypt from 'bcrypt';
export const hashPassword = (password: string): string => {
return bcrypt.hashSync(password, 10);
};
export const comparePassword = (password: string, hash: string): boolean => {
return bcrypt.compareSync(password, hash);
};
- You want to write unit tests for these functions, but running the actual hashing logic isn’t ideal for testing purposes.
Setting Up Vitest
To test these functions, you’ll need Vitest installed. You can add it to your project using npm:
npm install --save-dev vitest
Then, configure Vitest in your project by adding a vitest.config.ts
file if needed.
Mocking hashSync
in Vitest
To mock hashSync
properly, follow these steps:
Step 1: Create a Mock File
Create a mock file for the bcrypt
library. Vitest allows you to override modules using vi.mock
.
In your tests
directory, create a file named __mocks__/bcrypt.ts
:
export const hashSync = vi.fn(() => 'mocked-hash');
export const compareSync = vi.fn(() => true);
Step 2: Mock the Library in Tests
In your test file, mock the bcrypt
module before running your tests:
import { describe, it, expect, vi } from 'vitest';
import { hashPassword, comparePassword } from '../src/auth';
import bcrypt from 'bcrypt';
vi.mock('bcrypt');
describe('Auth Utility Functions', () => {
it('should hash a password', () => {
const password = 'my-secret-password';
const hash = hashPassword(password);
expect(bcrypt.hashSync).toHaveBeenCalledWith(password, 10);
expect(hash).toBe('mocked-hash');
});
it('should compare a password', () => {
const password = 'my-secret-password';
const hash = 'mocked-hash';
const isMatch = comparePassword(password, hash);
expect(bcrypt.compareSync).toHaveBeenCalledWith(password, hash);
expect(isMatch).toBe(true);
});
});
Step 3: Run the Tests
Run your tests using the Vitest command:
npx vitest
You should see your tests pass, with the mock functions being called as expected.
Debugging Common Issues
If you encounter issues while mocking hashSync
, consider the following tips:
- Check Your Mock Directory: Ensure the mock file is correctly placed in the
__mocks__
directory. - Verify Module Paths: Double-check the path used in
vi.mock
. It should match the import path in your source code. - Inspect Mock Implementations: If the default mock implementation doesn’t suit your needs, customize the behavior using
vi.fn
. - Use
clearMocks
: To prevent test interference, clear mocks between tests:vi.clearAllMocks();
Why Mocking Matters
Mocking hashSync
improves test efficiency and avoids unnecessary computation. It also ensures predictable test outcomes, as the actual hashing process can introduce variability.
By mocking, you focus on testing the logic of your code rather than the behavior of external dependencies.
Conclusion
Mocking the hashSync
function in Vitest is straightforward when you set up your mocks correctly. With the steps outlined above, you can efficiently test hashing logic in your TypeScript projects without compromising performance or reliability.
If you’re still facing challenges, consider exploring Vitest’s official documentation or libraries like ts-mockito for advanced mocking scenarios.